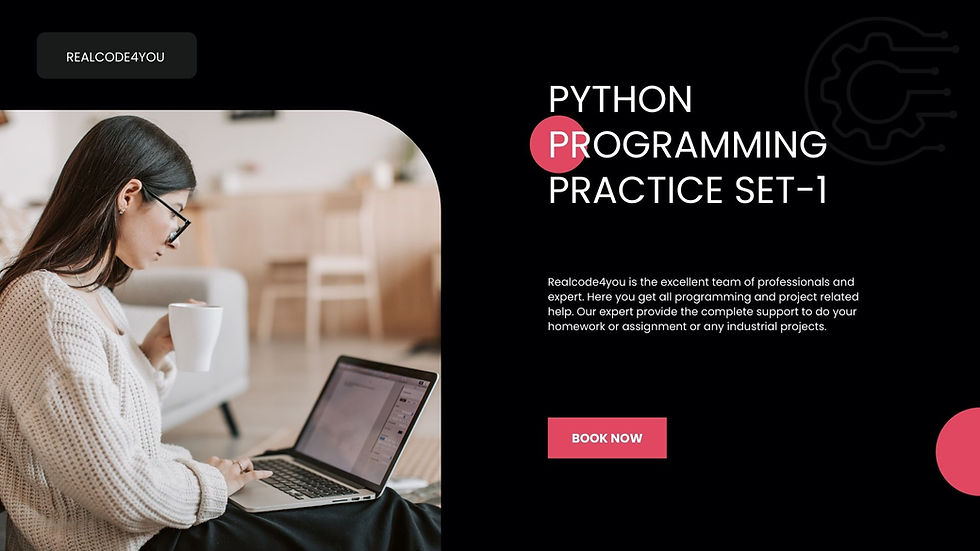
Question 1:
What are the differences between operators and values in the following?
*
'hello'
-87.8
-
/
+
6
Ans:
* : will perform multiplication operation on values
‘hello’ : is a string constant (made of sequenced order of characters)whose value do not change in the entire program.
-87.8 : is a float constant with negative value
- : is an operator which performs subtraction operation on values
/ : is an operator which performs division operation on values
+ : is an operator which performs addition operation on values
6 : is a integer constant
Question 2:
What is the difference between string and variable?
spam
'spam'
Ans:
spam is a variable which can take any value as int, string, float, etc and can be changed at any time
‘spam’is a string constant which remains same throughout .
Question 3:
Describe three different data forms.
Ans:
Assuming data forms to be data types,
Int-integer which takes integer values
float-float which takes float values
str-String which takes sequence of characters
Question 4:
What makes up an expression? What are the functions of all expressions?
Ans:
Expression is a combination of values, operators and assignment. The functions of all expressions is to be give a definite output once the expression is processed .
Question 5:
Assignment statements such as spam = 10 were added. What's the difference between a declaration and an expression?
Ans:
Declaration will assign a value to a variable .In this way, value assigned to variable determines the data type of variable.
Expression includes combination of values, operators and assignment.
Question 6:
After running the following code, what does the variable bacon contain?
bacon = 22
bacon + 1
Ans:
bacon=23 after running the code.
Question 7:
What should the values of the following two terms be?
'spam' + 'spamspam'
'spam' * 3
Ans:
’spam’+’spamspam’ =’spamspamspam’
‘spam’*3=’spamspamspam’
Question 8:
Why is it that eggs is a true variable name but 100 is not?
Ans:
eggs is a true variable name because it can be initialized to any data type and can take any value during the program
while 100 is well defined constant in any language and cannot be used to take data of any other form.
Question 9:
Which of the following three functions may be used to convert a value to an integer, a floating-point number, or a string?
Ans:
functions not given. However, below can be taken as an explanation
Two types of data type conversion in python
>implicit data type conversion: the type of data changes from one type to another without user involvement.
For example,
a=10
print(“the type of “a” is , type(a))
b=12.6
print(“the type of “b” is , type(b))
c=a+b
print(“c is{} and the type of “c” is , c ,type(c))
Output
type of a is int
type of b float
c is 22.6 and the type of c is float->As seen ,the type of “a” changes automatically to float
>explicit data type conversion: the type of data is changed manually in the program
For e.g s=”100001”
x= int(s,2) where 2 is the base to which the string will be converted
print (x)
Output
33->the string converted to base 2
Similarly, float(),hex(), oct() are other form to change data type to respective types.
Question 10:
What is the error caused by this expression? What would you do about it?
'I have eaten ' + 99 + ' burritos.'
Ans:
The first data is a string constant followed by integer constant and then followed by another string constant. The addition operation performed on two different data types.
As in this case , changing the type of integer constant”99” to string will be more logical
We can use , ‘I have eaten’+’99’+’burritos’ which would give an output as below
‘I have eaten 99 burritos’
Commenti