Python Interview Questions and Practice Set - 5 | Hire Python Expert to Get Help
- realcode4you
- May 23, 2022
- 3 min read
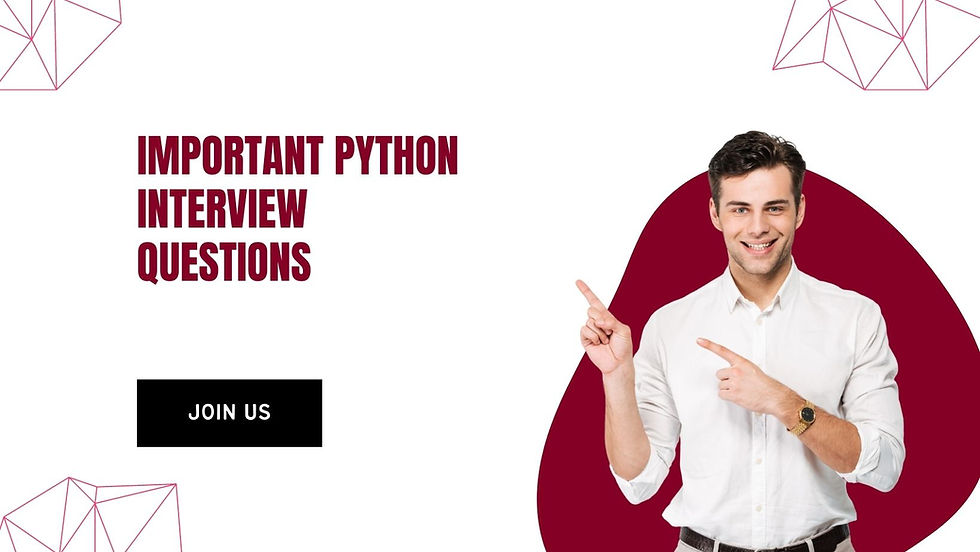
1. Is the Python Standard Library included with PyInputPlus?
Ans: PyInputPlus is not a part of the Python Standard Library, we must install it separately using Pip.
2. Why is PyInputPlus commonly imported with import pyinputplus as pypi?
Ans: You can import the module with import pyinputplus as pyip so that you can enter a shorter name when calling the module’s functions
3. How do you distinguish between inputInt() and inputFloat()?
Ans: The difference is in the data-type when you use the first one the program expects an integer value as input but in the latter it expects a float value i.e number containing a decimal
4. Using PyInputPlus, how do you ensure that the user enters a whole number between 0 and 99?
Ans: By using pyip.inputint(min=0, max=99)
5. What is transferred to the keyword arguments allowRegexes and blockRegexes?
Ans: A list of regex strings that are either explicitly allowed or denied
6. If a blank input is entered three times, what does inputStr(limit=3) do?
Ans: The function will raise RetryLimitException.
7. If blank input is entered three times, what does inputStr(limit=3, default='hello') do?
Ans: . The function returns the value 'hello'
8. To what does a relative path refer?
Ans: Relative paths are relative to the current working directory
9. What does an absolute path start with your operating system?
Ans: Absolute paths start with the root folder, such as / or C:\.
10. What do the functions os.getcwd() and os.chdir() do?
Ans: The os.getcwd() function returns the current working directory. The os.chdir() function changes the current working directory
11. What are the . and .. folders?
Ans: The . folder is the current folder, and .. is the parent folder
12. In C:\bacon\eggs\spam.txt, which part is the dir name, and which part is the base name?
Ans: C:\bacon\eggs is the dir name, while spam.txt is the base name.
13. What are the three “mode” arguments that can be passed to the open() function?
Ans: The string 'r' for read mode, 'w' for write mode, and 'a' for append mode
14. What happens if an existing file is opened in write mode?
Ans: An existing file opened in write mode is erased and completely overwritten
15. How do you tell the difference between read() and readlines()?
Ans: The read() method returns the file’s entire contents as a single string value. The readlines() method returns a list of strings, where each string is a line from the file’s contents.
16. What data structure does a shelf value resemble?
Ans: A shelf value resembles a dictionary value; it has keys and values, along with keys() and values() methods that work similarly to the dictionary methods of the same names
17. How do you distinguish between shutil.copy() and shutil.copytree()?
Ans: The shutil.copy() function will copy a single file, while shutil.copytree() will copy an entire folder, along with all its contents.
18. What function is used to rename files??
Ans: The shutil.move() function is used for renaming files, as well as moving them
19. What is the difference between the delete functions in the send2trash and shutil modules?
Ans: The send2trash functions will move a file or folder to the recycle bin, while shutil functions will permanently delete files and folders.
20.ZipFile objects have a close() method just like File objects’ close() method. What ZipFile method is equivalent to File objects’ open() method?
Ans: The zipfile.ZipFile() function is equivalent to the open() function; the first argument is the filename, and the second argument is the mode to open the ZIP file in (read, write, or append).
21. Create a programme that searches a folder tree for files with a certain file extension (such as .pdf or .jpg). Copy these files from whatever location they are in to a new folder.
Ans: import os, shutil
sourcePath = input(‘Enter the absolute path of the source folder: ‘) fileExtType = input(‘Enter the type of file to copy (such as .pdf or .jpg): ‘).lower() destPath = input(‘Enter the absolute path of the destination folder: ‘)
for foldername, subfolders, filenames in os.walk(sourcePath): for filename in filenames: if filename.lower().endswith(fileExtType): #print(foldername + ‘\\’ + filename) copySourcePath = os.path.join(foldername, filename) #print(copySourcePath) shutil.copy(copySourcePath, destPath) else: continue
Comentários