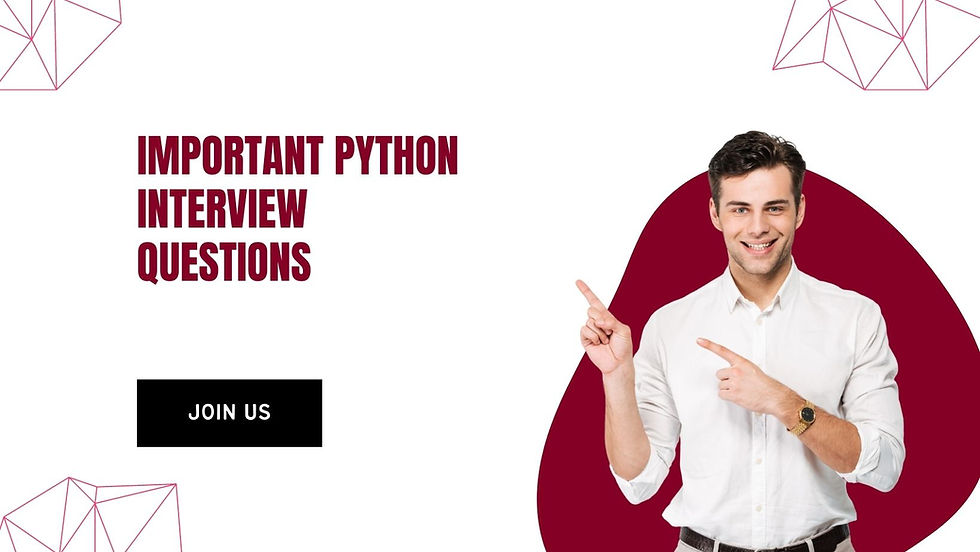
1. Why are functions advantageous to have in your programs?
Ans: Advantages of functions:- i. Avoid duplication and repetition of codes
ii. decompose complex programs into simpler ones.
iii. Improve clarity and readability of code.
iv. reduces chances of error
v. ease out modification of program
vi. information hiding
2. When does the code in a function run: when it's specified or when it's called?
Ans: the code in a function runs when it’s called.
3. What statement creates a function?
Ans: define function_name(arguments) : this statement creates a function
4. What is the difference between a function and a function call?
Ans: A function is procedure to achieve a particular result while function call is using this function to achieve that task.
5. How many global scopes are there in a Python program? How many local scopes?
Ans: The scope of a variable in python is that part of the code where it is visible.
Four types of scopes in python:
i>Local scope- variables declared inside function can only be utilized inside the function.
ii>Global scope-variables declared outside any other python variable scope and hence can be utilized anywhere in the program.
iii>Enclosed scope –variables which are not global or local, e.g,
>>> def red():
a=1
def blue():
b=2
print(a)
print(b)
blue()
print(a)
>>> red()
In this code, ‘b’ has local scope in function ‘blue’, and ‘a’ has nonlocal scope in‘blue’.
iv>Built in scope- The built-in scope has all the names that are loaded into python variable scope when we start the interpreter.
e.g,print(),id()
There are 1 global scope and 4 local scopes in python program.
6. What happens to variables in a local scope when the function call returns?
Ans:-The local variables are destroyed when the function call returns and memory occupied by them
are freed for any other variables.
7. What is the concept of a return value? Is it possible to have a return value in an expression?
Ans:- a return statement causes execution to leave the current subroutine and resume at the point in the code immediately after the instruction which called the subroutine, known as its return address. Yes, It is possible to have a return value in an expression, e.g,
>>> def greater_than_1(n):
... return n > 1
...
>>> print(greater_than_1(1))
False
>>> print(greater_than_1(2))
True
8. If a function does not have a return statement, what is the return value of a call to that function?
Ans:- None.
9. How do you make a function variable refer to the global variable?
Ans: We can use “global” keyword before the variable defined inside the function.
10. What is the data type of None?
Ans: None is a data type of its own NoneType) and only None can be (None). None keyword is an object, and it is a data type of the class NoneType
11. What does the sentence import areallyourpetsnamederic do?
Ans: This sentence imports the module “areallyourpetsnamederic “
12. If you had a bacon() feature in a spam module, what would you call it after importing spam?
Ans: by using spam.balcon()
13. What can you do to save a programme from crashing if it encounters an error?
Ans: error handling can be used to notify the user of why the error occurred and gracefully exit the process that caused the error
14. What is the purpose of the try clause? What is the purpose of the except clause?
Ans: The try block is used to check some code for errors i.e the code inside the try block will execute when there is no error in the program. Whereas the code inside the except block will execute whenever the program encounters some error in the preceding try block.
15. What exactly is []?
Ans: [] (foo-bar) is an empty list.
16. In a list of values stored in a variable called spam, how would you assign the value 'hello' as the third value? (Assume [2, 4, 6, 8, 10] are in spam.)
Ans: spam[2]=’hello’
Let's pretend the spam includes the list ['a', 'b', 'c', 'd'] for the next three queries.
17. What is the value of spam[int(int('3' * 2) / 11)]?
Ans: d. background: ‘3’*2=’33’ .int(‘33’)=33. 33/11=3.int(3)=3. Spam[3]=d.
18. What is the value of spam[-1]?
Ans: d. negative indices count from end.
19. What is the value of spam[:2]?
Ans: [‘a’,’b’]
Let's pretend bacon has the list [3.14, 'cat,' 11, 'cat,' True] for the next three questions.
20. What is the value of bacon.index('cat')?
Ans: 1. gives value of 1st occurrence.
21. How does bacon.append(99) change the look of the list value in bacon?
Ans: adds value at the end of list. Bacon=[3.14,’cat’,11,’cat’,True,99]
22. How does bacon.remove('cat') change the look of the list in bacon?
Ans: 1. Removes value of 1st occurrence. Bacon=[3.14 ,11,’cat’,True]
23. What are the list concatenation and list replication operators?
Ans: List concatenation operator: + and replication operator: *
24. What is difference between the list methods append() and insert()?
Ans: append() adds values at the end of list while insert() adds values anywhere inside list as specified indices
25. What are the two methods for removing items from a list?
Ans: remove() list method and del statement
26. Describe how list values and string values are identical.
Ans: Both lists and strings can be passed to len(), have indexes and slices, be used in for loops, be concatenated or replicated, and be used with the in and not in operators
27. What's the difference between tuples and lists?
Ans: Lists are mutable, i.e,values can be added, removed and changed from or in lists. Tuples are immutable. tuples uses () parenthesis and lists uses square [] brackets.
28. How do you type a tuple value that only contains the integer 42?
Ans: (42,).trailing comma is mandatory.
29. How do you get a list value's tuple form? How do you get a tuple value's list form?
Ans: tuple() and list() functions respectively.
30. Variables that "contain" list values are not necessarily lists themselves. Instead, what do they contain?
Ans: They contain references to list values
31. How do you distinguish between copy.copy() and copy.deepcopy()?
Ans: The copy.copy() function will do a shallow copy of a list, while the copy.deepcopy() function will do a deep copy of a list. That is, only copy .deepcopy() will duplicate any lists inside the list
Comments