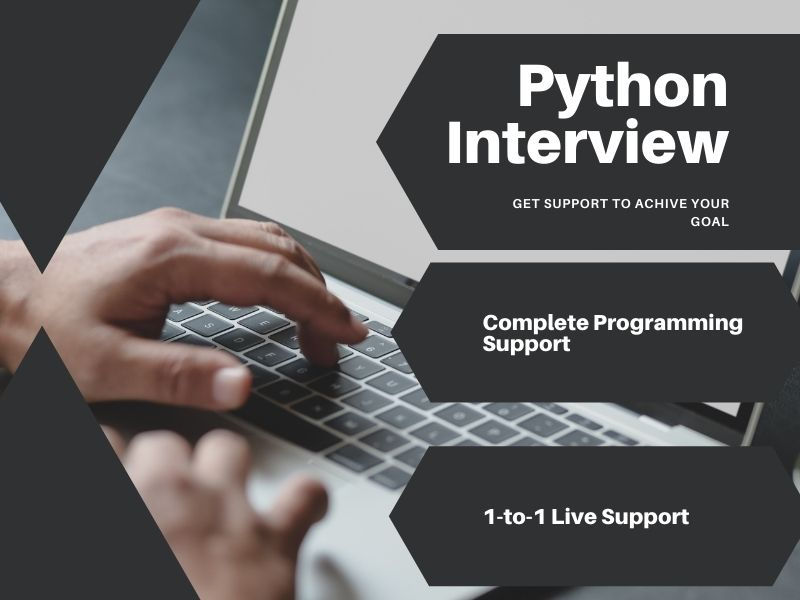
1. What does an empty dictionary's code look like?
Ans: Two curly brackets:{}
2. What is the value of a dictionary value with the key 'foo' and the value 42?
Ans: {‘foo’:42}
3. What is the most significant distinction between a dictionary and a list?
Ans: The items in dictionary are unordered and in a list are ordered.
4. What happens if you try to access spam['foo'] if spam is {'bar': 100}?
Ans: We get a KeyError error.
5. If a dictionary is stored in spam, what is the difference between the expressions 'cat' in spam and 'cat' in spam.keys()?
Ans: There is no difference. The in operator checks whether a value exists as a key in the dictionary
6. If a dictionary is stored in spam, what is the difference between the expressions 'cat' in spam and 'cat' in spam.values()?
Ans: 'cat' in spam checks whether there is a 'cat' key in the dictionary, while 'cat' in spam.values() checks whether there is a value 'cat' for one of the keys in spam.
7. What is a shortcut for the following code?
if 'color' not in spam:
spam['color'] = 'black'
Ans: spam.setdefault('color', 'black')
8. How do you "pretty print" dictionary values using which module and function?
Ans: pprint.pprint()
9. What are escape characters, and how do you use them?
Ans: Escape characters represent characters in string values that would otherwise be difficult or impossible to type into code.
10. What do the escape characters n and t stand for?
Ans: \n is a newline; \t is a tab.
11. What is the way to include backslash characters in a string?
Ans: The \\ escape character will represent a backslash character.
12. The string "Howl's Moving Castle" is a correct value. Why isn't the single quote character in the word Howl's not escaped a problem?
Ans: The single quote in Howl's is fine because we’ve used double quotes to mark the beginning and end of the string.
13. How do you write a string of newlines if you don't want to use the n character?
Ans: Multiline strings allow you to use newlines in strings without the \n escape character
14. What are the values of the given expressions?
'Hello, world!'[1]
'Hello, world!'[0:5]
'Hello, world!'[:5]
'Hello, world!'[3:]
Ans: The expressions evaluate to the following:
• 'e'
• 'Hello'
• 'Hello'
• 'lo world
15. What are the values of the following expressions?
'Hello'.upper()
'Hello'.upper().isupper()
'Hello'.upper().lower()
Ans: The expressions evaluate to the following:
• 'HELLO'
• True
• 'hello'
16. What are the values of the following expressions?
'Remember, remember, the fifth of July.'.split()
'-'.join('There can only one.'.split())
Ans: 1>[‘Remember,’,’remember,’,’the’,’fifth’,’of’,’july’]
2>’there-can-only-one’
17. What are the methods for right-justifying, left-justifying, and centering a string?
Ans: The rjust(), ljust(), and center() string methods, respectively
18. What is the best way to remove whitespace characters from the start or end?
Ans: The lstrip() and rstrip() methods remove whitespace from the left and right ends of a string, respectively
If you need support in interview or need answer of any questions related to python then comment in below comment section so we can help you.
We are also providing project help, assignment help and homework help with and affordable price.
Send your requirement details directly at:
realcode4you@gmail.com
Comments