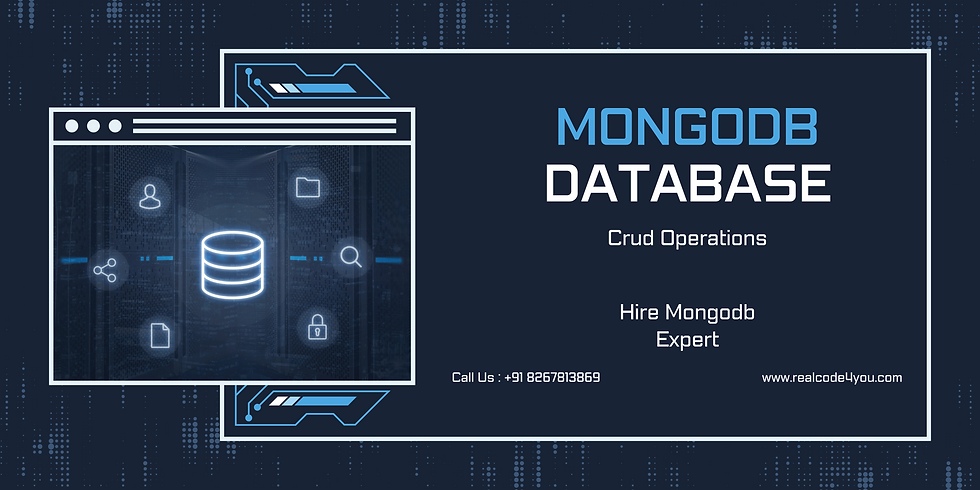
Software requirement
The following software is used in today’s workshop:
MongoDB 6.0
• Installation instructions:
Studio 3T
Here is an online tool that includes MongoDB and MapReduce, it has a 30 day trial but if you need more time you can also apply for a student license
CRUD operations
1. $nin
db.inventory.find({quantity:{$nin:[5, 15]}})
Selects all documents from the inventory collection where the quantity does not equal either 5 or 15
db.inventory.find({quantity:{$not:{$in:[5, 15]}}})
2. $exists
db.inventory.find({qty:{$exists:true}})
This query will select all documents in the inventory collection where the qty field exists.
3. $gt
db.inventory.find({quantity:{$gt:20}})
Select all documents in the inventory collection where quantity is greater than 20.
4. $lt
db.inventory.find({quantity:{$lt:20}})
Select all documents in the inventory collection where quantity is less than 20.
MongoDB can compare numbers in string format and it is done based on string comparison rules.
It compares Unicode code points of characters.
Ex.,:
"8" is U+0038 (56 in decimal)
"2" is U+0032 (50 in decimal)
5. Finding textual patterns (Contain)
db.inventory.find({context:{$regex:"Sydney"}})
db.inventory.find({context:{$regex:/Sydney/}})
db.inventory.find({context:/Sydney/})
Select all documents in the inventory collection where context contains “Sydney” word.
6. Finding textual patterns (Start with)
db.inventory.find({context:{$regex:"^Sydney"}})
db.inventory.find({context:{$regex:/^Sydney/}})
db.inventory.find({context:/^Sydney/})
Select all documents in the inventory collection where context starts with “Sydney” word.
7. Finding textual patterns (End with)
db.inventory.find({context:{$regex:"Sydney$"}})
db.inventory.find({context:{$regex:/Sydney$/}})
db.inventory.find({context:/Sydney$/})
Select all documents in the inventory collection where context ends with “Sydney” word
Display operations
1. Display contents of specific fields.
db.Tweets.find({query statement},{"display field1":1, "display field2":1})
2. Sort results according to values of a field.
db.Tweets.find({query statement}).sort({"sort field":1})
3. Count results.
db.Tweets.find({query statement}).count()
4. Return limited number of results.
db.Tweets.find({query statement}).limit(number)
Comments