Errors in code are often called bugs. Debugging is the process of finding and fixing those errors. The term 'bugs' has been used by engineers as far back as the 1840's to describe unexplained malfunctions. An actual computer problem caused by a dead moth in a relay in 1945 popularized the term for programmers.
Syntax Errors
Syntax Errors are errors that occur from not following the rules of the language. These errors generally prevent a program from running.
Some examples include:
Missing Semi-colon
typos
Missing Brackets
type mismatch
Debugging:
Syntax Errors are the easiest to find and fix. In the IntelliJ editor, there will either be a red squiggly line under a Syntax Error, or a word’s font may change to red. If you hold your mouse cursor over the squiggly line, you will get a popup box describing the problem.
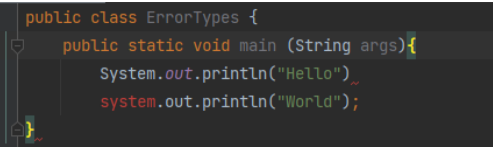
Runtime Errors
Runtime Errors occur when you no not anticipate certain input or situations.
For example:
invalid input
Array out of bounds
arithmetic Errors
infinite loop
With these types of errors your program will run and may seem to be working at first, but suddenly it will crash (or in the case of the infinite loop, get stuck).
Debugging:
Learning to read the stack trace is important for finding and fixing Runtime Errors. When your program crashes, you will get some sort of error message printed in the console. Two lines of the stack trace are especially important:
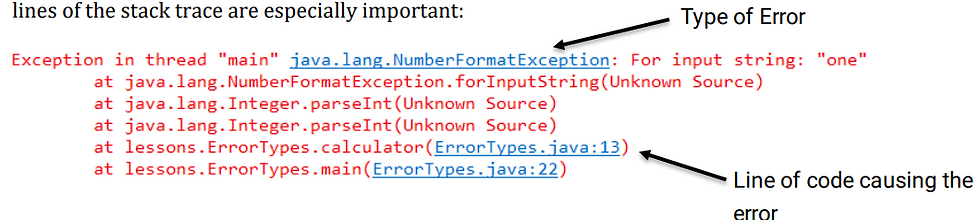
Logic Errors
Logic Errors are a type of Runtime Error. These are the hardest to find and fix because, quite often, your program runs without crashing. It simply produces the wrong result. For example, wanting to add two variables but coding answer = x – y; is a logic error.
Debugging:
Creating test cases is an important tool in fixing these bugs. Choose a set of inputs and calculate by hand what the output should be if everything was working properly
Once you know what the result should be, use:
1) Print Statements
Put temporary print statements are various points in your code
Print out the value of key variables, or just a word that indicates your program made it to a certain point
2) Debug Mode
Add a Breakpoint to a line in your code where you want to search for bugs
From the Run menu, choose Debug
By pressing the F5 key, you can move through your code one line at a time
3) Trace Tables
Sometimes there's just no substitute for writing the process out on paper
Trace through the code line by line, tracking the value of variables and output