IF Statements
Until now our programs have always done the same thing each time they were run. But to be truly useful programs need to change based on user input. To accomplish this in programming we use IF Statements.
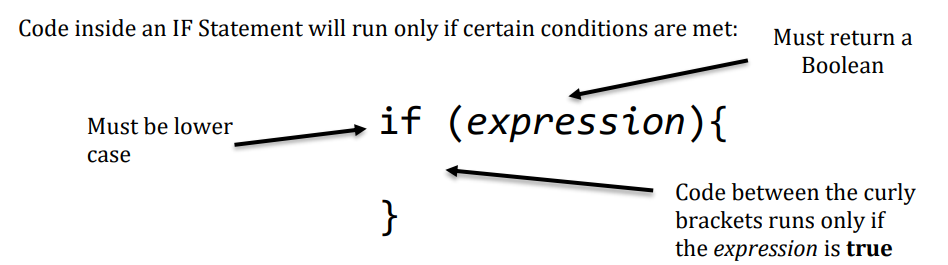
Example:
if (x == 5){
System.out.println("This only prints if x is 5");
System.out.println("This also only prints if x is 5");
}
System.out.println("This prints no matter what x is");
Else Statement
An Else Statement acts as a catch-all. If none of the previous conditions were met, whatever code is in the Else statement will run.
x = 7;
if (x == 5){
System.out.println ("if x is not 5, this will not print");
} else{
System.out.println ("This code runs because the previous condition was not met");
}
Else If Statements
In Java you can use else if to check additional conditions if the previous ones have failed. You can have as many else if statements in an IF statement as you want.
Note: As soon as one condition is met, Java skips to the end of the IF statement without checking any of the others.
Example:
x = 6;
if (x == 5){
System.out.println ("x is not 5, this will not print");
} else if (x==6){
System.out.println ("x does = 6, this line prints!");
} else{
System.out.println ("This code never runs because the previous condition was met");
}
Comparison Operators
Comparison operators are used to check the size of values compared to each other. They will return a Boolean value of true or false.
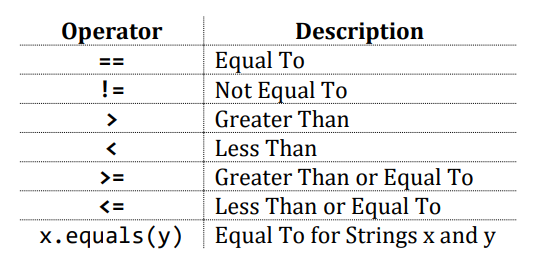
Logical Operators
There are three logical operators: and, or and not
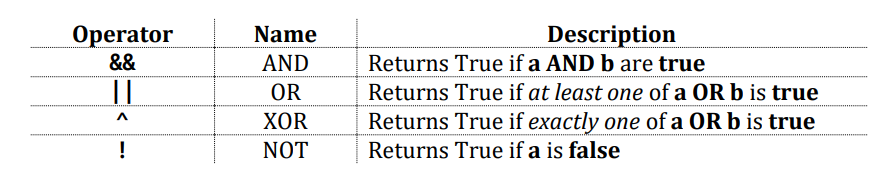